The Volume Depth Sampler is a regular class that allows you to raytrace a 3D render texture and read voxel data from 3D render texture.
It needs proper initialization with a target 3D render texture and raytrace data (iterations and threshold).
Remember to call 'Dispose' to release sensitive resources.
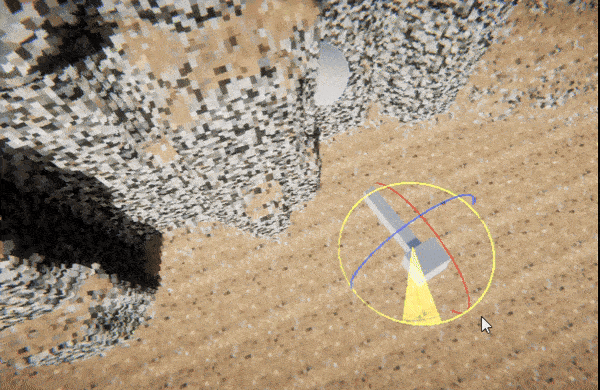
The Depth Sampler enables you to sample and read pixel data from a target RT3D. Two methods can be called:
SampleVolumeDepth and
SampleVolumePixel.
-
SampleVolumeDepth returns a boolean parameter indicating whether the ray hit the raytraced voxel and outputs the world hit position of the voxel.
-
SampleVolumePixel outputs a vec3 with the actual hit voxel value (red channel), material index (green channel) and an extra channel value (blue channel).
These methods can be utilized to precisely sample the target volume box with tex3D data and place objects in the scene.
You can utilize the Volume Depth Sampler in your own C# script, dispatching all the parameters manually, or you can leverage the example content provided by Raymarcher.
In the example content, there's a script named
'RMSample_VolumeBrushLocator' that already encompasses all the functionality for placing an object on the target volume.
This script works closely with the
'RMSample_VolumeVoxelPainterFPS' script, which automatically creates a Volume Voxel Painter.
You can either use this script or create a custom script that initializes the Volume Voxel Painter and sets up the Volume Brush Locator.
The
Volume Brush Locator essentially positions a 'brush' object on the target volume box and is primarily used as a 'brush' in voxel painting.
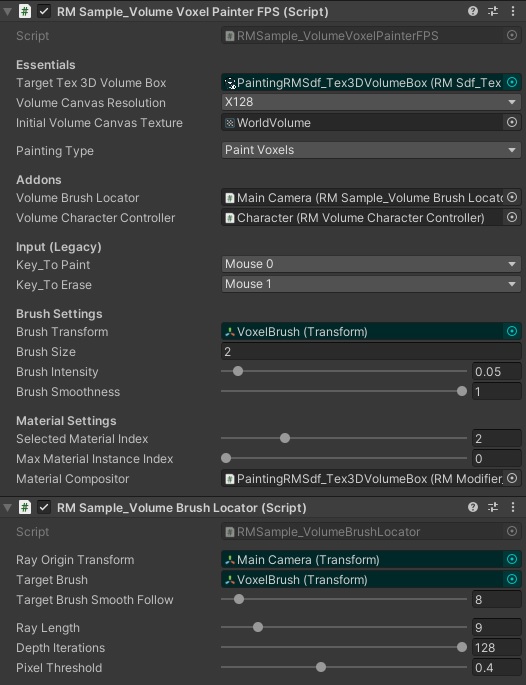