The
RMSdfObjectBase class serves as a foundational class for scripts defining 'objects' within the Raymarcher renderer.
These objects are specific types of Signed Distance Functions (SDFs) that leverage mathematical formulas to approximate the shape of the desired SDF object.
RMSdfObjectBase contains an interface implementation of
ISDFEntity, defining common parameters for all SDF entities, including modifiers.
For a visual representation of the data structure of Raymarcher objects and modifiers, refer to the image below:
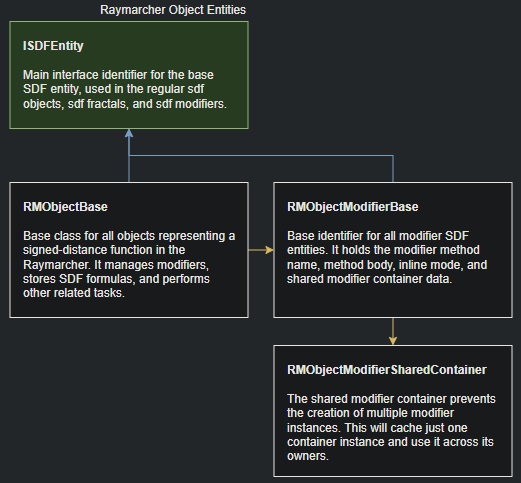
Inherit from this class to define your custom SDF object within the Raymarcher renderer.
Once you create a gameObject in Unity with a mono class inheriting from
RMSdfObjectBase, the Raymarcher will automatically integrate the object into the Object Buffer.
If the Object Buffer detects changes, the converter will prompt for Raymarcher's recompilation.
It is required to accurately define the properties that the
RMSdfObjectBase class expects to be overridden.
List of abstract methods and properties essential for proper SDF object definitions:
Each Raymarcher SDF object shares common values, although some of these values are active and visible only in specific Raymarcher Render Types.
For example, when the render type is set to Performant, the object's material won't be utilized, as the Performant render type supports global materials only.
List of public fields and properties shared among all Raymarcher SDF objects: